L'architecture de Jahia en bref
- Un CMS (
Content Management System ) polyglotte, où les développeurs peuvent déployer des modules écrits en JavaScript ou Java - Une CDP (
Customer Data Platform ), basée sur Apache Unomi, avec une interface utilisateur pour l'analyse, la personnalisation et la segmentation - Une intégration parfaite avec Elasticsearch pour la recherche
plain-text
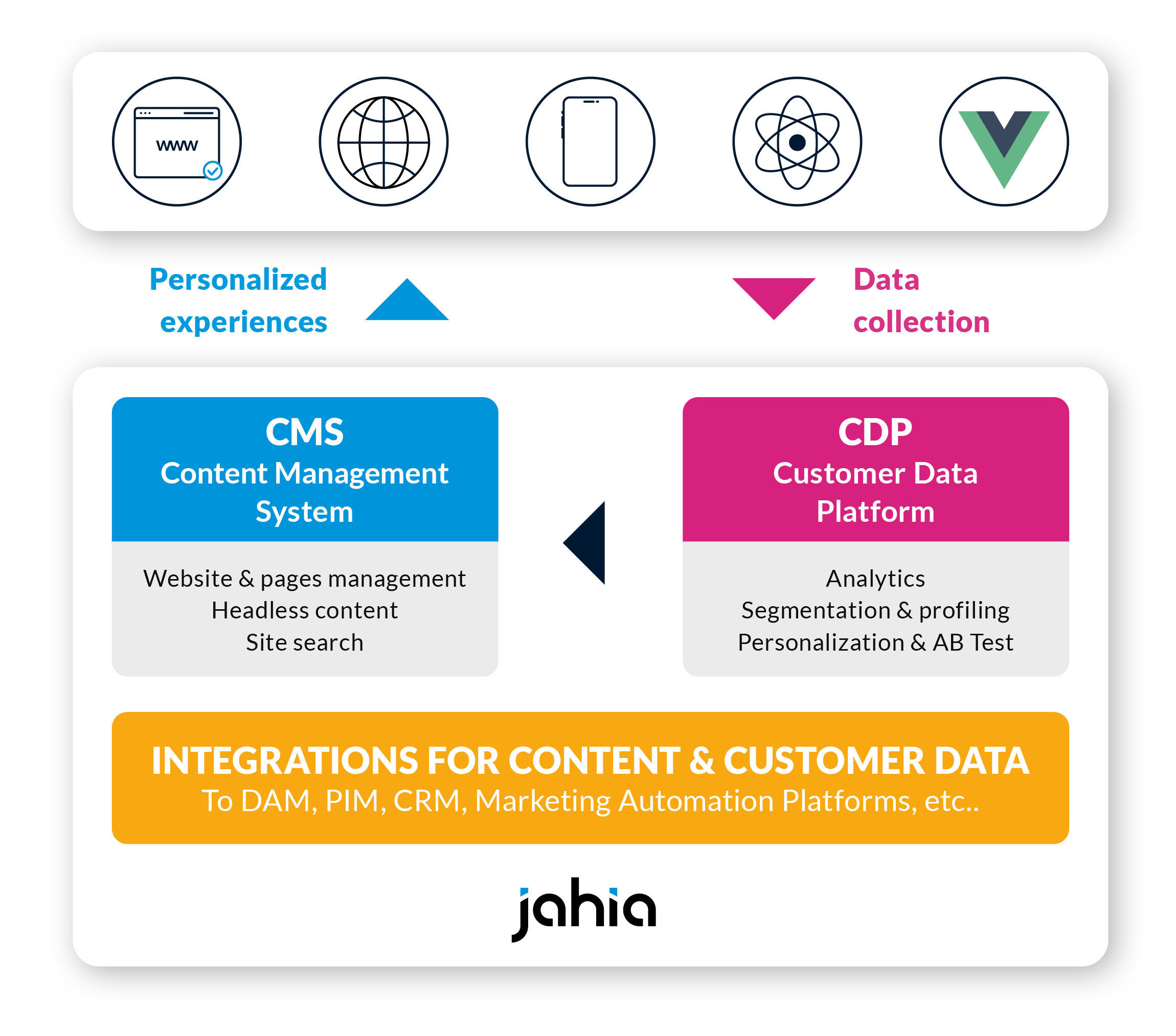
Architecture du CMS Java et du CMS Javascript de Jahia
CMS Headless Hybride (head-optional)
Une approche pragmatique du Headless basée sur une expérience éprouvée
Jahia est un CMS hybride, notre solution est API first, mais pas API-only. Jahia propose une approche pragmatique et peut produire des pages HTML.
- API GraphQL pour appeler du contenu (JSON)
- Possibilité d'appeler des fragments HTML
- Recherche full-text
- Provisionnement API pour déployer le code à l'aide de chaînes CI/CD
- Customer Data APIs publiques pour la collecte de données
- APIs de données client protégées pour la gestion des données
Modulith : Une alternative éprouvée aux microservices
Intégrez dans notre plateforme tout ce que vous souhaitez, pour couvrir les besoins les plus exigeants
- Extension du CMS Jahia avec des modules Java, qui sont des bundles OSGi, comme avec des modules Javascript, qui sont des paquets NPM.
- L'OSGi et son implémentation Apache Karaf disponible dans Jahia offre :
- déploiement à chaud,
- gestion des dépendances,
- isolation.
- Déclarez toute logique personnalisée, y compris :
- Des services Java et des actions,
- Des extensions d'APIs,
- Du JSP, des balises et des filtres si vous utilisez la couche de présentation,
- Des permissions,
- Des extensions de l'interface utilisateurs.
Intégrations de contenu
Plusieurs modèles d'intégration pour intégrer DAM, PIM ou tout autre système hébergeant du contenu ou des actifs.
- Le fournisseur de données externes (External Data Provider) fournit un framework Java permettant l'intégration de systèmes externes en tant que fournisseurs de contenu. Tout contenu externe peut être géré et rédigé comme s'il était hébergé dans Jahia.
- Des services Java personnalisés peuvent être créés pour faire appel à des systèmes externes.
- L'interface utilisateur de Jahia peut être fortement étendue pour réaliser des intégrations directement à partir du front-end.
- Des connecteurs prêts à l'emploi sont disponibles pour Widen, Akeneo, Siteimprove et d'autres encore.
Une plateforme de données client intégrée (CDP), le meilleur complément d'un CMS
Notre infrastructure de données clients est basée sur Apache Unomi
- Public customer data API for current visitor tracking (1st party cookie based)
- GraphQL API pour le contenu personnalisé & ; AB Tests
- Création de profils à l'aide d'événements de connexion
- Analyse intégrée et personnalisable, utilisant Elasticsearch Kibana
- Private / admin customer data REST API for data aggregation, custom event type declaration, rules and actions deployment (API REST pour l'agrégation de données, la déclaration de types d'événements personnalisés, le déploiement de règles et d'actions)
- Modèle de données simple et puissant : profils d'accès, sessions et événements. Stockez et accédez aux données de n'importe quel formulaire, enquête, quiz, entonnoir en temps réel.
Intégrations des données clients
Jahia DXP est livré avec "StackConnect", un connecteur complet à Workato. Workato est une Integration- Platform-As-A-Service (iPaas) de premier plan, et les connecteurs sont inclus dans l'abonnement à la DXP Jahia Cloud.
- Intégrations no-code grâce à Workato
- 1000+ connecteurs avec des plateformes CRM, Marketing Automation et email marketing
- Intégrations bidirectionnelles pour enrichir le profil des visiteurs ou pousser des données collectées à partir de comportements utilisateurs ou de formulaires
- Des intégrations entièrement personnalisées utilisant les règles Apache Unomi et les actions Groovy sont également possibles
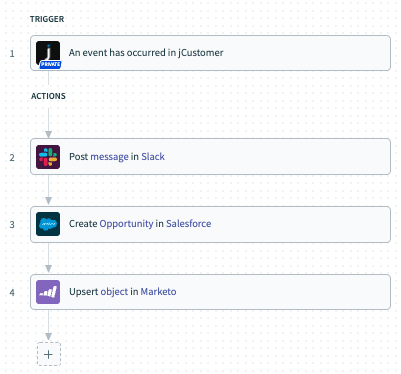
Authentification, sécurité et conformité
Cloud & sécurité
Jahia Cloud, DXP-as-a-Service
- UI de gestion Cloud en libre-service : créer, dupliquer, sauvegarder, restaurer ou faire évoluer n'importe quel environnement en quelques clics
- Plateform-as-a-Service (PaaS) avec séparation stricte des données entre les comptes : profitez de la flexibilité d'un environnement dédié sans payer pour un service en gestion personnalisée, et sans compromettre la sécurité de vos environnements
- Une infrastructure toujours à jour : grâce à des correctifs de sécurité déployés rapidement et silencieusement pour vous, votre DXP exécute toujours la dernière version
- Opérations sans temps d'arrêt (0-downtime) : déploiements blue/green en quelques clics
- Bénéficiez d'un support 24/7/365 et d'infrastructures hautement disponibles répliquées dans plusieurs centres de données en conformité ISO 27001 et HIPAA à tous les niveaux
Authentication & permissions
Tout ce que vous pouvez attendre d'une plateforme d'entreprise
- Solutions d'authentification complètes pour avec un support intégré pour se connecter à LDAP, SSO, SAML & OAuth
- Rôles et autorisations granulaires pour définir exactement ce à quoi les utilisateurs et les groupes peuvent accéder sur l'ensemble de la plateforme, pour un site ou même pour une page ou un élément de contenu
- La même logique est également exploitée pour gérer les expériences authentifiées et donner un accès spécifique aux pages, aux API et au contenu à vos clients ou fournisseurs
- Intégration complète et transparente avec les caches front-end et back-end
Vue d'ensemble des modules d'authentification de Jahia:
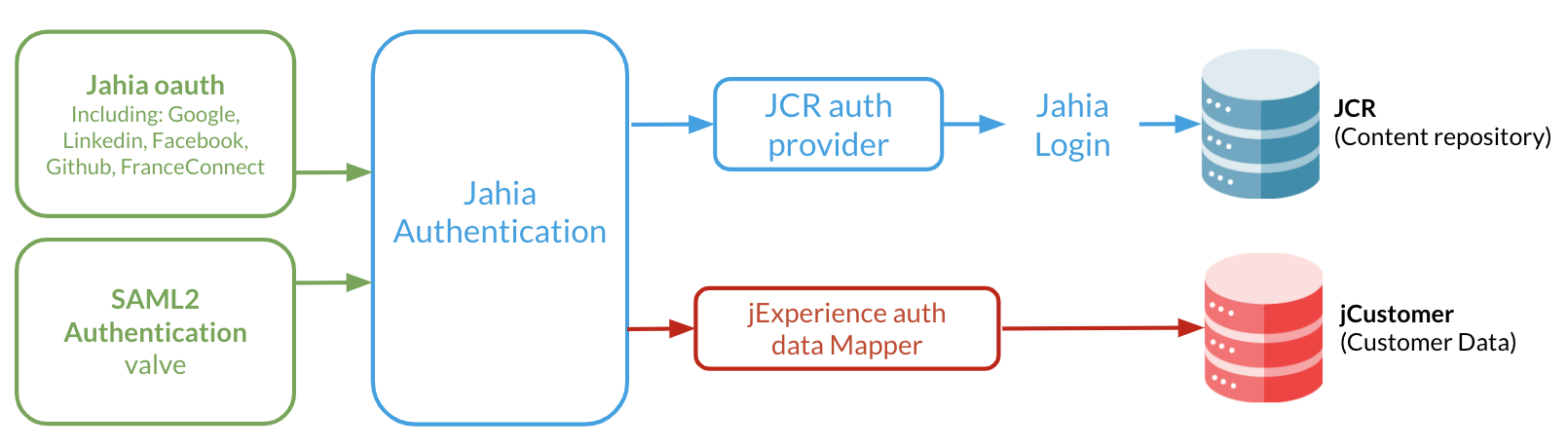
Un véritable engagement en faveur de l'open source
Jahia entretient depuis longtemps des relations amicales avec l'Open Source.
- Jahia CMS est open core, la plupart du code que nous produisons est sous licence Apache ou MIT.
- Jahia a initié Apache Unomi, et contribue toujours fortement au projet.
- Il est possible d'essayer Jahia en utilisant notre édition communautaire et nos images Docker publiques.